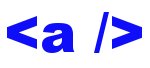
This is because sites that offer API access don’t want people to flood their resources with bots that can take away access from other users or just cause havoc.
Most of the time the execution is no more then 1 request per second. Now in many scripts you may find that there are multiple accesses to an outside API this is true for website affiliates that run a Amazon Store or people that access free API resources.
In PHP there are a few different ways to delay your script the most common is the built in sleep function. The Sleep function will allow you to delay the processing of your app by a number of whole seconds that you request.
PHP Sleep Example
[php]</div>
<div><code> <?php
// current time
echo date(‘h:i:s’) . "n";
// sleep for 10 seconds
sleep(10);
// wake up !
echo date(‘h:i:s’) . "n";
?> </code></div>
</div>
<div>[/php]
<div><code> <?php
// current time
echo date(‘h:i:s’) . "n";
// sleep for 10 seconds
sleep(10);
// wake up !
echo date(‘h:i:s’) . "n";
?> </code></div>
</div>
<div>[/php]
As you can see this script will echo the time and then wait 10 seconds then echo it again.
When you run the script the output of the first echo is likely to be delayed but when you compare the two times you will see that they are 10 seconds apart.
PHP usleep Function
The php function usleep is just like the sleep function but it allows you fine control over the time delay.
In usleep you define the number of microseconds that the script should wait during execution.
[php]</div>
<div>
<div><code> <?php
// Current time
echo date(‘h:i:s’) . "n";
// wait for 2 seconds
usleep(2000000);
// back!
echo date(‘h:i:s’) . "n";
?> </code></div>
<div>[/php]
<div>
<div><code> <?php
// Current time
echo date(‘h:i:s’) . "n";
// wait for 2 seconds
usleep(2000000);
// back!
echo date(‘h:i:s’) . "n";
?> </code></div>
<div>[/php]
The PHP function time_nanosleep
nanosleep is similar to sleep and usleep but the delay is in nanoseconds.
[php]time_nanosleep ( int <tt>$seconds</tt> , int <tt>$nanoseconds</tt> )[/php]
The PHP Function time_sleep_until
The last function that we will look at is time_sleep_until this function will allow you to specify an exact time that the script should wake back up.
[php]bool time_sleep_until ( float <tt>$timestamp</tt> )[/php]
This can be tricky especially if your $timestamp happens to be in the past. This could happen under heavy load so calculating a start and wakeup time in your script that is also within the maximum execution time may throw back errors.
For more information about all of these PHP Functions you can visit php.net.